The electronics section of the HAL interface dies not require a tremendous amount of work. It consists of an Adafruit Feather M0 single board micro-controller with an Adafruit Feather Wing Musicmaker plugged on top. A ztx450 transistor connects to one of the GPIO pins to control the 10mm LED “eye”. The circuit is so simple that I have not bothered to make a custom pcb. Instead, I am using a small piece of circuit strip board often known as Veroboard.
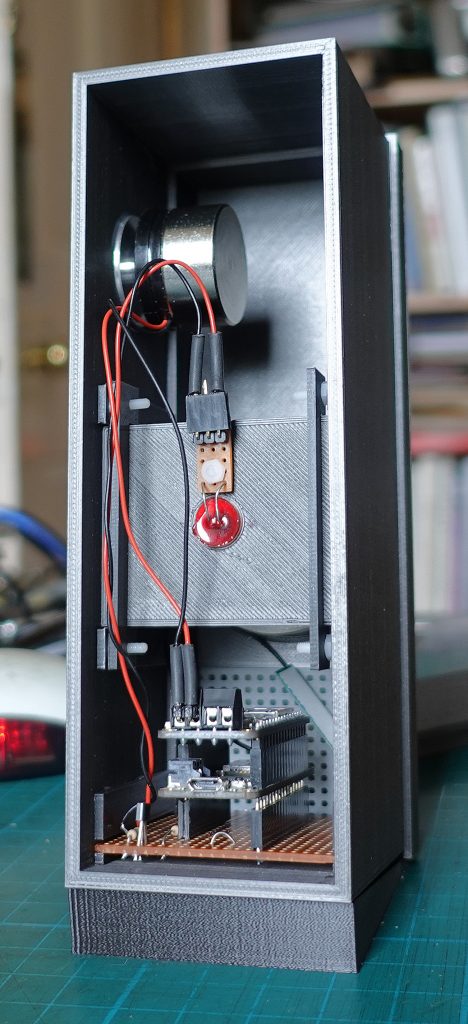
To plug the Feather into the circuit, Solder two 0.1” pitch female header sockets to the strip board, one 12 pin, the other 16 pin. To cut the header, pull out one of the pins with a small pair of pliers and use a fine saw to cut though the plastic where the pin used to be. Something like an Xacto or similar saw used for model making is good for this. You need to cut the tracks in between the rows of Feather pins. You can get a special tool for this but a 4mm drill bit mounted in a handle for ease of use just turned by hand in one of the holes in the strip board in the appropriate place will do the job.
The LED for the eye is soldered to a tiny piece of strip board fixed to the lens rear 3D print with a 2.5mm nylon nut and bolt. The connection is made using right angle 0.1 pitch male header and female header as shown in the picture left. I have used heat shrink to protect the wires where they are soldered to the female header. (I always use adhesive lined shrink tube.)
The device top left in the case is an Adafruit medium size surface transducer. This is in effect a loudspeaker which depends on the surface it rests on being vibrated by the transducer and hence vibrating the air in contact with it producing sound (as long as someone’s there to hear it!) As long as the surface it’s attached to is reasonably springy, the sound produced is surprisingly good (to my ears anyway). Putting the transducer on the body of an acoustic guitar, for example, produces a warm, rich tone. The 3D-printed side of the case, less so but still ok. I saw this advertised and thought I would try it out instead of a conventional speaker set behind the grille just for the sake of interest! The transducer is Araldited to the side of the case, by the way.
The amplifier on the Musicmaker is stereo. I don’t know whether it automatically mixes left and right tracks when only one speaker is connected but for this application it makes no odds. There is room in the case for a second transducer but at the time of writing, it is “out of stock” (shortage in time of plague? – I am writing this April 2020!
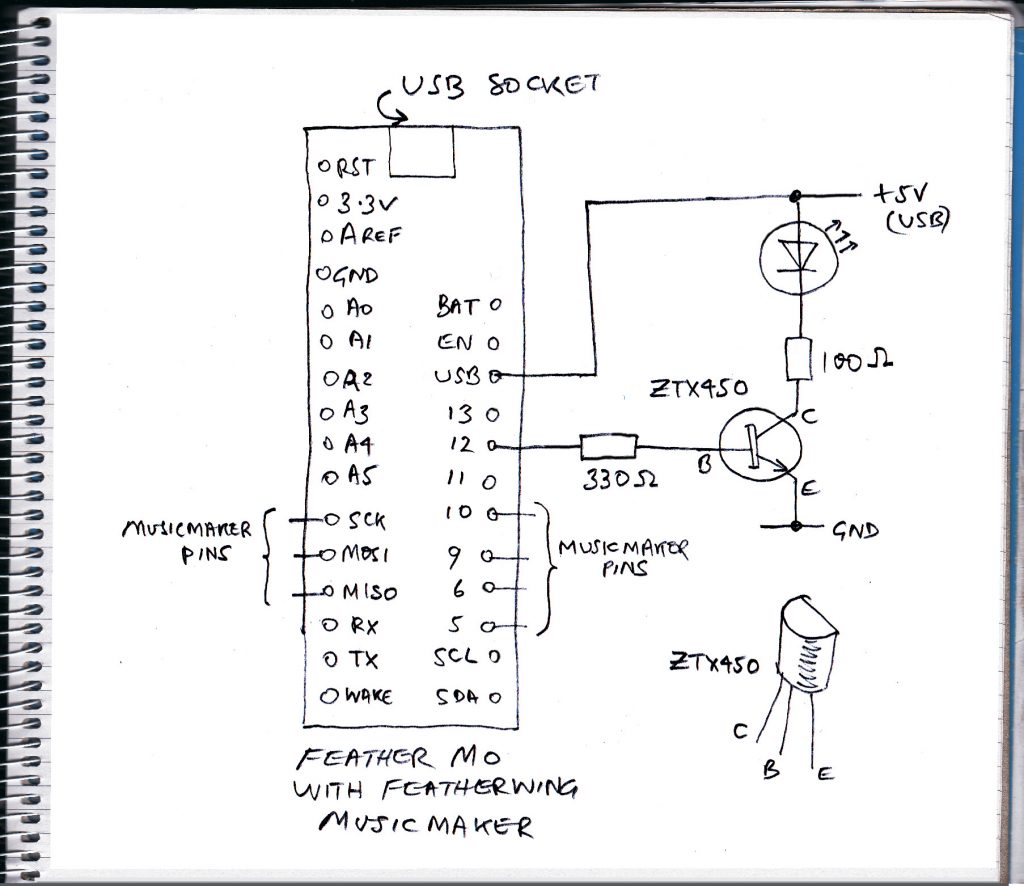
Above, behold the simplicity of the circuit! Seven pins are reserved for Musicmaker use, the rest are free and could be used to get inputs from, say, a motion detector or, as outputs, trigger all manner of stuff you might want to have done. As mentioned in the introduction, the Feather could be controlled by WiFi or directly through serial via the usb port. At present the software randomly plays one of 36 clips with random gaps in between. At the same time the brightness of the LED waxes and wanes a little to convey extra “sentience”
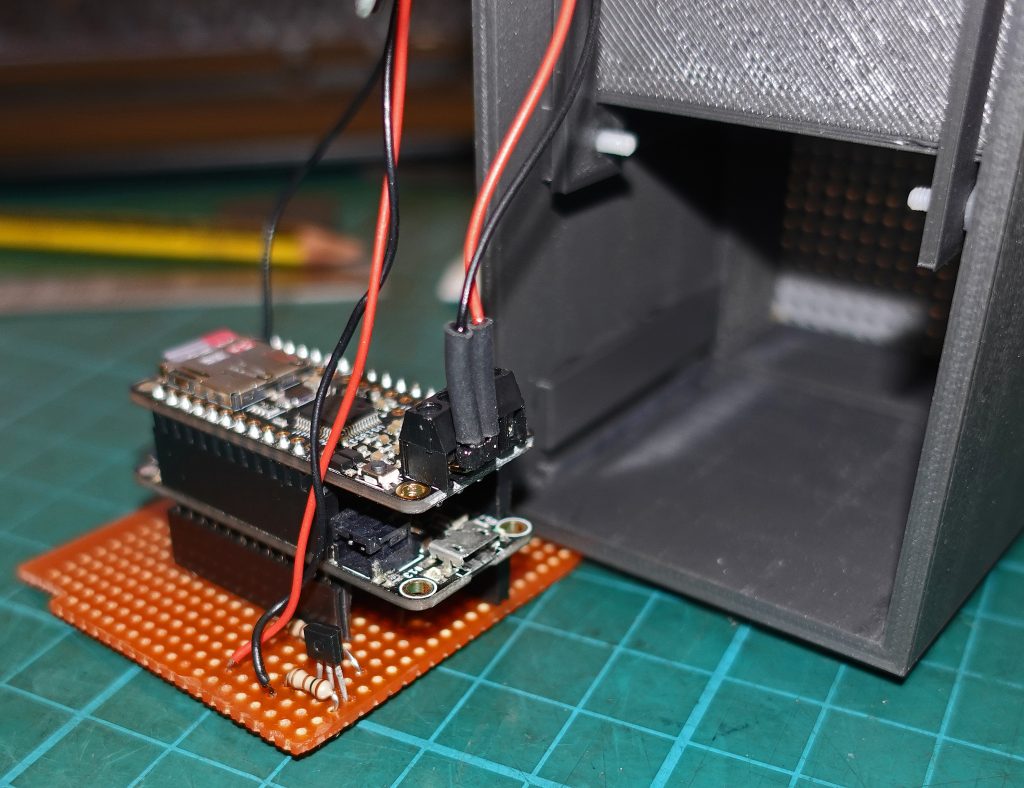
In the picture above you can see the SD card on which the clips of HAL’s voice are stored. Near the front is the ztx450 transistor which drives the red LED. The supplied terminals for the speaker wires do not retain small stranded wire well. I have soldered the wires onto right-angled male headers, protected by shrink fit tubing, which work much better.
HAL Software
The software is adapted from one of the Adafruit examples to play mp3 clips using the Adafruit Musicmaker. The clips are played in interrupt mode which means that anything else can be going on in the main loop of the program and not be stopped while the clips play.
The program detects when the current clip finishes and then generates a random number which is used to select the next clip. There is a greater range of random numbers than there are clips so there is a random interval between clips. You could make the random numbers “more random” by using noise from an unused analogue pin to seed the random function as described in the Arduino documentation. When HAL’s voice is not playing, a clip of “cabin ambient sounds” plays continuously.
At present all that happens apart from playing the clips is that the red LED’s brightness waxes and wanes slightly to convey more “sentience”. However, sensors to detect a person in front of the interface, control over WiFi or input via serial on the usb could be easily incorporated (I think!)
// HAL comms
// 10.3.20
// halcoms3
// adapted from Adafruit MusicMaker example
// include SPI, MP3 and SD libraries
#include <SPI.h>
#include <SD.h>
#include <Adafruit_VS1053.h>
// These are the pins used
#define VS1053_RESET -1 // VS1053 reset pin (not used!)
#define VS1053_CS 6 // VS1053 chip select pin (output)
#define VS1053_DCS 10 // VS1053 Data/command select pin (output)
#define CARDCS 5 // Card chip select pin
#define VS1053_DREQ 9 // VS1053 Data request, ideally an Interrupt pin
byte t;
Adafruit_VS1053_FilePlayer musicPlayer =
Adafruit_VS1053_FilePlayer(VS1053_RESET, VS1053_CS, VS1053_DCS, VS1053_DREQ, CARDCS);
void setup() {
pinMode(12, OUTPUT); // pin controls LED eye
Serial.begin(9600);
delay(500);
musicPlayer.begin();
SD.begin(CARDCS);
// Set volume for left, right channels. lower numbers == louder volume!
musicPlayer.setVolume(0,0);
// If DREQ is on an interrupt pin we can do background audio playing
musicPlayer.useInterrupt(VS1053_FILEPLAYER_PIN_INT); // DREQ int
}
//------------------------------------------------------------
void loop() {
// file names of mp3 clips are /1.mp3, /2.mp3 etc.
t = int(random(1,100)); // number > number of clips adding a random gap
//wait for player to stop playing then select a random clip
if(!musicPlayer.playingMusic){
if(t==1){
musicPlayer.startPlayingFile("/1.mp3");
}
if(t==2){
musicPlayer.startPlayingFile("/2.mp3");
}
if(t==3){
musicPlayer.startPlayingFile("/3.mp3");
}
if(t==4){
musicPlayer.startPlayingFile("/4.mp3");
}
if(t==5){
musicPlayer.startPlayingFile("/5.mp3");
}
if(t==6){
musicPlayer.startPlayingFile("/6.mp3");
}
if(t==7){
musicPlayer.startPlayingFile("/7.mp3");
}
if(t==8){
musicPlayer.startPlayingFile("/8.mp3");
}
if(t==9){
musicPlayer.startPlayingFile("/9.mp3");
}
if(t==10){
musicPlayer.startPlayingFile("/10.mp3");
}
if(t==11){
musicPlayer.startPlayingFile("/11.mp3");
}
if(t==12){
musicPlayer.startPlayingFile("/12.mp3");
}
if(t==13){
musicPlayer.startPlayingFile("/13.mp3");
}
if(t==14){
musicPlayer.startPlayingFile("/14.mp3");
}
if(t==15){
musicPlayer.startPlayingFile("/15.mp3");
}
if(t==16){
musicPlayer.startPlayingFile("/16.mp3");
}
if(t==17){
musicPlayer.startPlayingFile("/17.mp3");
}
if(t==18){
musicPlayer.startPlayingFile("/18.mp3");
}
if(t==19){
musicPlayer.startPlayingFile("/19.mp3");
}
if(t==20){
musicPlayer.startPlayingFile("/20.mp3");
}
if(t==21){
musicPlayer.startPlayingFile("/21.mp3");
}
if(t==22){
musicPlayer.startPlayingFile("/22.mp3");
}
if(t==23){
musicPlayer.startPlayingFile("/23.mp3");
}
if(t==24){
musicPlayer.startPlayingFile("/24.mp3");
}
if(t==25){
musicPlayer.startPlayingFile("/25.mp3");
}
if(t==26){
musicPlayer.startPlayingFile("/26.mp3");
}
if(t==27){
musicPlayer.startPlayingFile("/27.mp3");
}
if(t==28){
musicPlayer.startPlayingFile("/28.mp3");
}
if(t==29){
musicPlayer.startPlayingFile("/29.mp3");
}
if(t==30){
musicPlayer.startPlayingFile("/30.mp3");
}
if(t==31){
musicPlayer.startPlayingFile("/31.mp3");
}
if(t==32){
musicPlayer.startPlayingFile("/32.mp3");
}
if(t==33){
musicPlayer.startPlayingFile("/33.mp3");
}
if(t==34){
musicPlayer.startPlayingFile("/34.mp3");
}
if(t==35){
musicPlayer.startPlayingFile("/35.mp3");
}
if(t==36){
musicPlayer.startPlayingFile("/36.mp3");
}
if(t > 36){
// cabin noise plays between clips
musicPlayer.startPlayingFile("/noise.mp3");
}
}
//---------------------------------------------
//brightness of led changes for extra "sentience"
for(int i = 50; i <= 255; i++){
analogWrite(12, i);
delay(10);
}
for(int i = 255; i >= 50; i--){
analogWrite(12, i);
delay(10);
}
}
//--------------------------------------------------
Recording the clips
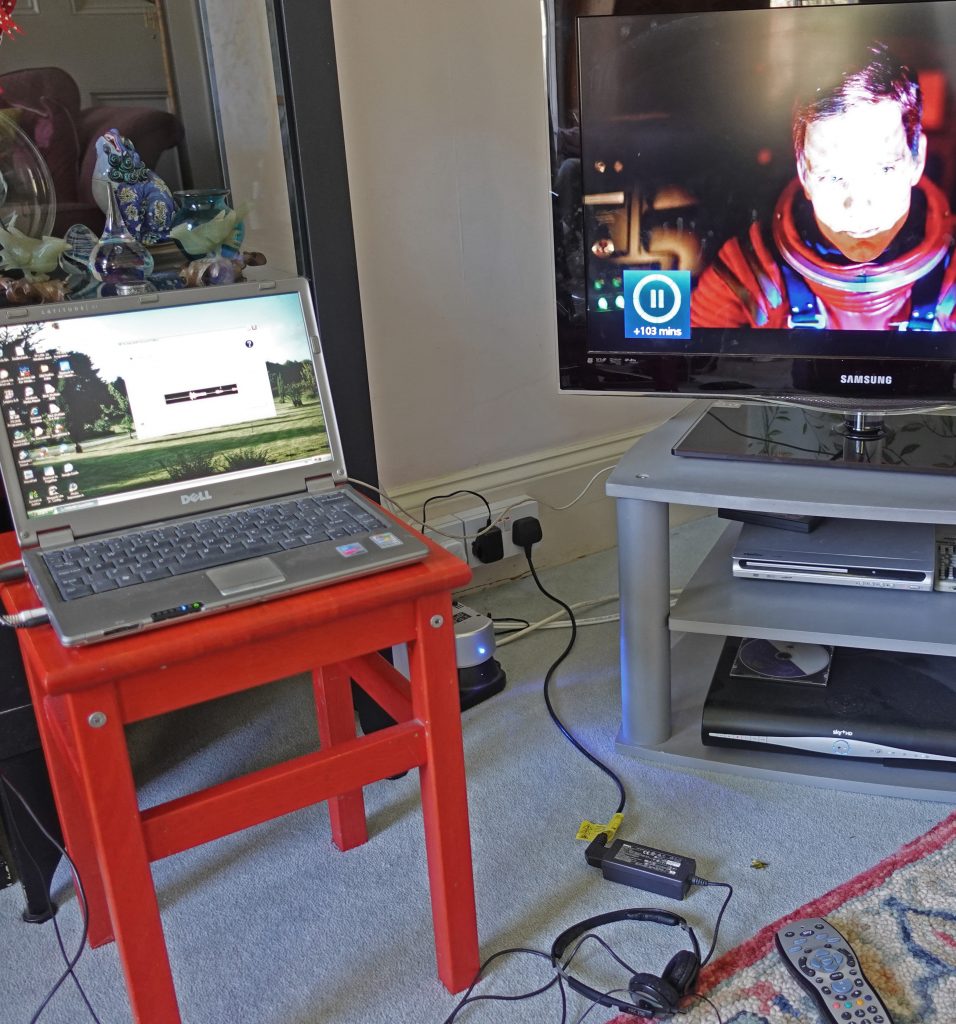
I’m sure this is ok from a copyright point of view for personal use only but don’t quote me!
Anyway, I recorded 2001 on my digital box whose brand shall be nameless and the recorded the clips by connecting the audio out on the box/tv with the mic/in on our old XP laptop running an audio recorder called Polderbits (now defunct owing to the sad death of the author of the software). It had the advantage of being able to save in mp3 format whereas Audacity had to make do with ogg. Now Audacity can export in mp3, that’s the best free software to use for this sort of job. When I got the raw audio onto my PC, I used Audacity to cut it up into bits for HAL’s clips.